Web DevelopmentTop ReactJS Best Practices for Frontend Teams
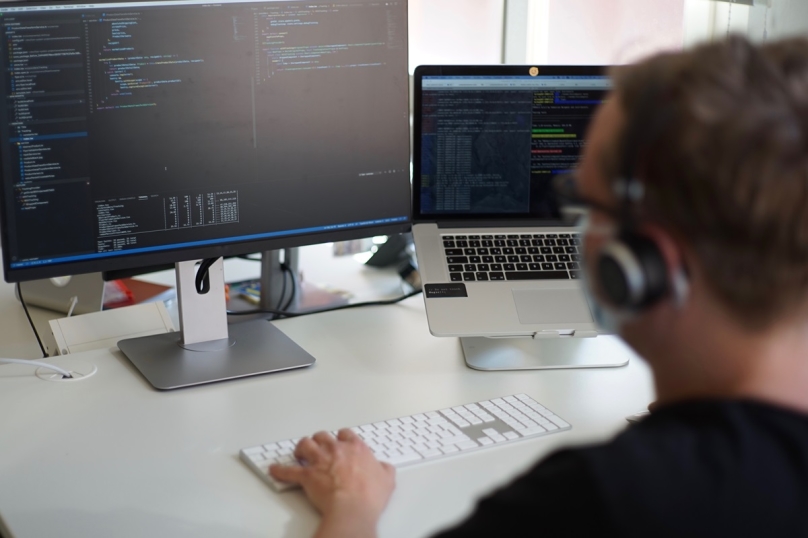
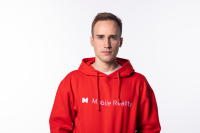
Marcin Sadowski
CTO @ JS and Web3 Expert
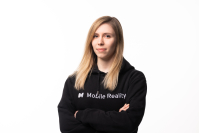
Magda Dembna
Frontend & Mobile Team Leader
Table of contents
Share the article
Introduction
React JS has firmly established itself as a front-runner for building modern web applications. Adhering to React JS best practices is crucial for teams aiming to leverage the full potential of this powerful library, ensuring that their projects are not only efficient but also scalable and maintainable. These best practices encompass a range of strategies, from structuring projects and managing state to implementing component designs, optimizing performance and keeping clean code. This article serves as a comprehensive guide to these essential practices, tailored specifically for ReactJS developers and frontend development teams who are dedicated to creating high-quality, robust React applications during the web development process.
Understanding React Basics
Grasping the core fundamentals of React is essential for any developer embarking on building dynamic and responsive web applications. This foundational knowledge empowers developers to write better code and lays the groundwork for effectively adopting advanced React JS best practices. At Mobile Reality, we emphasize a thorough understanding of key advantages of React concepts such as JSX, components, props, state, and the React lifecycle, which are crucial for crafting efficient and maintainable React applications.
JSX and Virtual DOM
React uses JSX for templating instead of regular JavaScript. JSX is a syntax extension that looks similar to HTML and allows developers to write HTML structures in the same file as JavaScript code. This blending of markup with logic helps React developers visualize user interfaces as they code, enhancing the development process's efficiency and clarity.
The Virtual DOM is another React concept that significantly optimizes web performance. It is a lightweight copy of the actual DOM (Document Object Model), and it allows React to do minimal DOM manipulation by batching changes in memory and updating the actual DOM as efficiently as possible. Understanding how React updates the Virtual DOM is key to writing performance-optimized React code.
Components and Prop Types
Components are the building blocks of any React application. They allow developers to break down the UI into reusable, independent pieces that can be handled separately. There are two types of components in React—functional components and class components. As noted in the Mobile Reality practices, functional components are now more commonly used due to their simplicity and the power of React hooks. It enables functional components to manage state and lifecycle events, which is traditionally only possible with class components.
Props (short for properties) are how data flows down from parent to child components. They are read-only and should be considered immutable within the child component. At Mobile Reality, we ensure that all components that require custom data or behavior receive it through props, maintaining a unidirectional data flow that is easier to debug and understand.
State Management and Lifecycle Methods
State management is crucial in React to handle data that changes over time. The useState
hook allows functional components to hold and set state, whereas class components use the this.state
and this.setState
approach. Effective state management ensures that the React application responds to user inputs and system events through a predictable data lifecycle, which is essential for developing interactive applications.
Lifecycle methods are special methods in class components that run according to the lifecycle of components. These methods include componentDidMount
, componentDidUpdate
, and componentWillUnmount
. They are used for operations that need a clean up or should be run after the component has rendered to the DOM. Understanding these methods is important for performing side effects, fetching data, and direct DOM manipulation.
Organizing Your Project Structure
Creating an effective project structure is crucial for efficient development and maintenance, especially in larger or more complex React applications. Based on the practices outlined in the "Frontend Practices of Mobile Reality" document, let’s detail a project organization strategy that aligns with these guidelines and enhances both scalability and maintainability.
High-Level Directory Layout
The document emphasizes a clear and modular directory layout, which is crucial for managing large-scale applications seamlessly. Here's a refined look at how the directories are structured at Mobile Reality:
/my-app
|-- /public
|-- /src
| |-- /api
| |-- /components
| |-- /constants
| |-- /hooks
| |-- /pages
| |-- /utils
| |-- /views
|-- /tests
|-- package.json
|-- README.md
Detailed Breakdown
1. /public
Houses static assets like images,
index.html
, and fonts that are publicly served without processing by Webpack.
2. /src: The core of the application's code.
/api: Contains all functions and utilities related to API interactions, such as Axios instances, API route configurations, and service definitions.
/components: Dedicated to reusable UI components. This directory should be organized further into subdirectories where each component, like buttons or modals, has its folder containing its JSX and CSS modules.
/constants: Stores constant values used across the application, such as route paths and configuration settings.
/hooks: For custom React hooks that encapsulate shared logic across components, such as fetching data or interacting with the Context API.
/pages: Only necessary in projects using Next.js or similar frameworks that require page-based routing; otherwise, it’s merged with views.
/utils: Utility functions that are non-component specific, such as date formatters or currency converters.
/views: Represents the components that are tied directly to routes. This directory can be thought of as the 'pages' in a traditional React setup and is critical for delineating different parts of the application.
3. /tests
A directory to maintain all unit and integration tests, ideally mirroring the structure of
/src
for easy navigation and consistency.
Component Organization within Directories
Each component directory should contain all related files, including its test files, styles, and any sub-components it exclusively uses. This co-location strategy ensures that components are self-contained and easily manageable. Example of Component Directory Structure:
/src
|-- /components
|-- /Button
|-- index.jsx
|-- Button.test.js
|-- Button.module.css
Naming Conventions
Following the guide in your PDF:
- Files and directories are named using PascalCase for components and camelCase for non-component files.
- Tests are placed alongside their corresponding components, promoting easier maintenance and cohesive updates when components evolve.
Additional Practices
- Views as Patterns: As emphasized in the document, views are considered the backbone of the application architecture. They should clearly represent the app’s various UI states and are typically aligned with routes.
- Nested Routes: Organizing nested routes and shared components such as navigation bars within the views or components folder helps keep related functionalities bundled together, promoting better scalability and reusability.
By aligning our project structure with these practices described in the Mobile Reality PDF, developers can ensure that the application remains organized and scalable, facilitating both individual development tasks and team collaborations effectively. This structure not only supports the current application needs but also accommodates future growth and expansion seamlessly.
Ready to start development of your ReactJS application?

Or contact us:
Component Design Patterns
In React, the choice between functional and class components is significant and can influence your application's simplicity, maintainability, and performance. Understanding the distinctions and appropriate use cases for each type of component is crucial for any React developer.
Functional Components
Functional components are JavaScript functions that accept props as arguments and return React elements. They are the preferred choice in modern React development, especially since the introduction of hooks in React 16.8, which allowed functional components to manage state and side effects.
Advantages of Functional Components:
- Simplicity: Without the need for this
keyword, functional components have a simpler syntax and are easier to read and test.
- Hooks: Functional components leverage hooks such as useState
, useEffect
, and custom hooks, making them capable of performing the same tasks as class components.
- Performance: They are generally less bulky than class components and may have performance benefits in certain cases due to optimizations like React.memo.
Example of a Functional Component:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
In addition to state management with useState
, functional components can handle lifecycle events using useEffect
, providing a powerful way to encapsulate functionality in a clear and concise manner.
Class Components
Class components are more traditional in React. They require ES6 classes to create and offer more features than functional components, especially before hooks were introduced. Class components are useful when dealing with complex state logic and providing richer event handling by leveraging lifecycle methods such as componentDidMount
, componentShouldUpdate
, and componentWillUnmount
.
Advantages of Class Components:
- Lifecycle Methods: They provide explicit declaration of lifecycle methods, which can be more manageable for handling complex interactions and side effects in the component.
- State Management: Historically, they were the only way to handle state in React components, though this has changed with hooks.
Example of a Class Component:
class Welcome extends React.Component {
constructor(props) {
super(props);
this.state = {name: props.name};
}
componentDidMount() {
console.log('Component did mount!');
}
render() {
return <h1>Hello, {this.state.name}</h1>;
}
}
Despite these features, class components have some downsides:
- Verbosity: They can be more verbose and complex due to syntax and the necessity of understanding this
context.
- Optimization Issues: Sometimes they can lead to less optimized performance compared to functional components, especially if not properly managed.
Choosing Between Functional and Class Components
When deciding which type of component to use, consider the following:
- Project Requirements: If the project is heavily based on hooks and functional programming paradigms, use functional components.
- Complexity: For applications that benefit from explicit use of lifecycle methods or when migrating legacy applications that heavily use class components, continuing with class components might be practical.
Overall, the trend in React development is moving towards functional components due to their simplicity and the power of hooks. However, understanding both types remains important as legacy codebases and certain complex scenarios might still benefit from the structured approach offered by class components. This balanced understanding ensures developers can choose the best tool for their specific needs, enhancing both development efficiency and application performance.
Effective State Management
Effective state management is crucial for the smooth functioning and maintainability of React applications. At Mobile Reality, we have adopted specific practices and structures to ensure that state management enhances the performance and scalability of our applications.
State Management with Hooks
In our projects, we heavily utilize React hooks, such as useState
for managing local state within components and useEffect
for handling side effects that depend on state changes. This approach keeps our components functional and concise, making them easier to test and maintain. Example of Using Hooks for State Management:
import React, { useState, useEffect } from 'react';
function Counter() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
});
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
This example is illustrative of how we manage component-level state effectively, leveraging hooks for both state and lifecycle management.
Context API for Global State
For global state management across different components, we leverage React's Context API. This approach is particularly beneficial in avoiding prop drilling, thereby keeping the component tree cleaner and more manageable. We often combine Context with useReducer when dealing with more complex state logic that involves multiple sub-values or when the next state depends on the previous one. Example of Context with useReducer:
import React, { createContext, useReducer, useContext } from 'react';
const initialState = {count: 0};
const CountContext = createContext();
function countReducer(state, action) {
switch (action.type) {
case 'increment':
return {count: state.count + 1};
case 'decrement':
return {count: state.count - 1};
default:
throw new Error();
}
}
function CountProvider({ children }) {
const [state, dispatch] = useReducer(countReducer, initialState);
return (
<CountContext.Provider value={{state, dispatch}}>
{children}
</CountContext.Provider>
);
}
function Counter() {
const { state, dispatch } = useContext(CountContext);
return (
<div>
Count: {state.count}
<button onClick={() => dispatch({type: 'increment'})}>+</button>
<button onClick={() => dispatch({type: 'decrement'})}>-</button>
</div>
);
}
In this setup, the CountProvider
wraps parts of our application where the state needs to be accessible, supporting a clean and modular approach to state management.
Best Practices for State Management
At Mobile Reality, we emphasize several best practices for state management:
Keep state local where possible: Elevate state to a global context only when necessary.
Minimize re-renders: Use memoization and shouldComponentUpdate wisely to prevent unnecessary re-renders.
Immutable state updates: Treat state as immutable, always returning new objects or arrays when updating state.
By following these practices, we ensure that our applications are both performant and maintainable, capable of scaling effectively as they grow in complexity and user base. These state management strategies are an integral part of our development standards, reflecting our commitment to quality and best practices in React development.
Coding and Naming Conventions
Adhering to consistent coding and naming conventions is critical for ensuring that a React project is maintainable, scalable, and easily understandable by all team members. At Mobile Reality, we have established specific standards that align with industry best practices and enhance the collaboration within our development teams. These conventions not only improve readability but also streamline the development process.
Component Naming and Structure
In our React projects, component names are pivotal for maintaining clarity. We use PascalCase for all React component names, which is a common naming convention that distinguishes components from regular JavaScript functions. This approach is in line with the React community's standards and helps in quickly identifying component files within the project. Example of Component Naming:
File:
UserProfile.jsx
Component Declaration:
function UserProfile() { ... }
Components are stored in individual folders, named after the component itself, which might include additional files like CSS modules, tests, and any subcomponents. This structure ensures that all relevant files for a component are grouped together, promoting a clean and organized file system. Example of Component Structure:
/src
|-- /components
|-- /UserProfile
|-- UserProfile.jsx
|-- UserProfile.test.js
|-- UserProfile.module.css
Prop Naming and Usage
For props, we follow a clear and descriptive naming convention using camelCase. When naming props, it’s important to reflect their purpose and function, making the code self-explanatory and minimizing the need for additional comments.
Example of Prop Naming:
In a
Button
component, a prop controlling whether the button is disabled might be namedisDisabled
.
Moreover, when it comes to passing props, we emphasize the importance of destructuring props in the component function parameter. This practice not only makes the component cleaner but also makes it easier to see at a glance which props are being used. Example of Prop Destructuring:
function UserProfile({ userName, userAge }) {
return (
<div>
<p>Name: {userName}</p>
<p>Age: {userAge}</p>
</div>
);
}
JavaScript Specifics and React Code Practices
In terms of JavaScript usage within React, we maintain a consistent code style across all our projects. This includes consistent use of arrow functions for inline functions and functional components, unless a class component is necessary. We also enforce the use of template literals over string concatenation for better readability and maintainability. Example of Using Arrow Functions and Template Literals:
const WelcomeMessage = ({ name }) => <h1>Welcome, {`${name}`}!</h1>;
CSS and Inline Styles
While managing styles, we prefer using CSS modules or styled-components to ensure styles are scoped to components without causing side effects elsewhere in the application. Inline styles are used sparingly and only when necessary for dynamic styling purposes. Example of CSS Module:
import styles from './UserProfile.module.css';
function UserProfile({ userName }) {
return <div className={styles.userProfile}>Username: {userName}</div>;
}
Enforcing Best Practices
To ensure these conventions are consistently applied, we use tools like ESLint and Prettier. These tools help automate and enforce a consistent coding style, catching common errors and formatting code according to our predefined rules.
By adhering to these coding and naming conventions, we not only keep our codebase clean and well-organized but also enhance the developer experience and productivity. These practices are essential for supporting the scalability and maintainability of our React applications at Mobile Reality.
React Code Optimization Techniques
Optimizing React applications is critical for improving performance and user experience, especially as applications scale in complexity and size. At Mobile Reality, we focus on several key strategies to ensure that our React projects are not only performant but also resource-efficient. These strategies align with our commitment to writing clean React code and leveraging React's built-in features to their fullest potential.
Minimizing Re-renders
One of the fundamental ways to enhance performance in React applications is to minimize unnecessary re-renders. This can be achieved by ensuring that components only update when truly necessary. Utilizing React.memo
for functional components is a strategy we employ to prevent re-renders caused by unchanged props. For class components, implementing shouldComponentUpdate
provides a way to control the rendering behavior based on changes in state or props. Example of React.memo:
const MyComponent = React.memo(function MyComponent(props) {
/* render using props */
});
Lazy Loading Components
React's lazy loading feature, utilized via React.lazy
and Suspense
, is particularly useful for large applications that include components with heavy dependencies or components that are not immediately necessary during the initial load. This technique allows us to split the code into smaller chunks and load them only when they are needed, effectively reducing the initial load time and smoothing out the user experience. Example of Lazy Loading:
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
Efficient Data Fetching
Strategically managing when and how data is fetched in a React application can drastically improve performance. We recommend utilizing hooks like useEffect
for fetching data upon component mount, and where feasible, delaying or paginating data requests to avoid overwhelming the user and system resources. Employing techniques such as SWR (stale-while-revalidate) or React Query can also help in managing server state with effective caching and background updates.
Code Splitting
In addition to lazy loading individual components, React supports code splitting at the route level. This allows developers at Mobile Reality to divide the application into separate bundles which can be loaded on-demand as a user navigates through the application. This practice significantly improves the initial load time by only loading the user-seen components. Example of Route-based Code Splitting:
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
const HomePage = lazy(() => import('./routes/HomePage'));
const AboutPage = lazy(() => import('./routes/AboutPage'));
function App() {
return (
<Router>
<Suspense fallback={<div>Loading...</div>}>
<Switch>
<Route exact path="/" component={HomePage} />
<Route path="/about" component={AboutPage} />
</Switch>
</Suspense>
</Router>
);
}
Using Immutable Data Structures
Maintaining immutability in the state is crucial for optimizing React applications. Immutable data structures ensure that the React's diffing algorithm can quickly determine if re-renders are necessary. This approach avoids accidental mutations and ensures consistent behavior across the application.
Security Best Practices
Ensuring the security of React applications is paramount to safeguarding user data and maintaining trust. At Mobile Reality, we implement a comprehensive set of security best practices that align with industry standards to mitigate potential vulnerabilities. These practices not only protect the application but also enhance its reliability and the safety of its users.
Validate Props with PropTypes
One of the fundamental practices we follow at Mobile Reality is validating props using PropTypes. This ensures that components receive the correct type of data, which can prevent bugs and potential security flaws that arise from unexpected data types. By enforcing type checking, we can catch errors early in the development process, making the code more robust and secure. Prop Validation Example:
import PropTypes from 'prop-types';
function UserProfile({ username, age }) {
return (
<div>
<p>Username: {username}</p>
<p>Age: {age}</p>
</div>
);
}
UserProfile.propTypes = {
username: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
};
Sanitize Inputs to Prevent Injection Attacks
Sanitizing user inputs is critical to prevent injection attacks, such as SQL injection or XSS (Cross-Site Scripting). When handling forms or any component that receives user-generated content, we ensure that inputs are sanitized either on the client side before submission or preferably on the server side before processing. This involves stripping out any potentially dangerous characters or HTML tags that could be used to execute malicious scripts. Example of Input Sanitization:
function sanitizeInput(input) {
return input.replace(/<script.*?>.*?<\/script>/gi, '');
}
Implement Secure HTTP Headers
To enhance the security of our React applications, we implement secure HTTP headers using middleware in our backend services. Headers such as Content-Security-Policy (CSP) help prevent XSS attacks by controlling the resources the client is allowed to load. Other headers like X-Frame-Options and X-XSS-Protection provide additional layers of security.
Use HTTPS for Data Transmission
All data transmissions in our applications are conducted over HTTPS to ensure that data sent between the client and server is encrypted. This prevents attackers from intercepting sensitive information like passwords or personal data. Using HTTPS is a critical security measure for protecting our applications against man-in-the-middle attacks.
Manage Authentication and Authorization Effectively
Authentication and authorization are key areas where security needs to be tight. We use modern authentication mechanisms like JWT (JSON Web Tokens) or OAuth to manage user sessions securely. These tokens are stored securely in HTTPOnly cookies or other secure storage mechanisms to prevent access from malicious scripts. Example of Secure Token Storage:
localStorage.setItem('AuthToken', JSON.stringify(token)); // Avoid if possible
Note: Storing sensitive information in localStorage should generally be avoided due to its susceptibility to XSS attacks. Prefer secure methods like HTTPOnly cookies or secure contexts.
Regularly Update Dependencies
Keeping all project dependencies up to date is another crucial practice. This helps protect against vulnerabilities found in older versions of libraries and tools. We regularly update our React projects and check for any security patches or updates that need to be applied.
Handling Props Effectively
Managing props effectively is key to building small, reusable components. Use PropTypes for type checking and set defaultProps for optional props to ensure components behave predictably. Default Props Example:
UserProfile.defaultProps = {
avatar: 'defaultAvatar.png',
};
React Hooks: Usage and Benefits
Hooks offer a way to use state and other React features without writing a class. useState
, useEffect
, and custom hooks allow for cleaner and more modular code. Using Hooks:
const [count, setCount] = useState(0);
useEffect(() => {
document.title = You clicked ${count} times;
});
Frontend Development Insights: Mastering ReactJS and VueJS
Are you fascinated by the evolving landscape of web and frontend development, particularly in ReactJS and VueJS? At Mobile Reality, we are eager to share our rich expertise and insights in these cutting-edge technologies. Uncover the challenges we navigate, the innovative strategies we employ, and the groundbreaking solutions we deliver in ReactJS and VueJS development. Immerse yourself in our curated selection of articles, each a deep dive into aspects of these powerful frontend frameworks:
- Micro frontend Architecture Guideline
- Pros & Cons of TypeScript In Web Development
- Creating your own streaming app with WebRTC
- A* Pathfinding in React JS Web Development
- Angular vs React JS - Ultimate Comparison
- NextJS Server Side Rendering Framework Guideline
- GatsbyJS: The Ultimate Web Development Guideline
- Progressive Web Apps Development: Guideline for CTOs
- Master Vue JS with These 5 Real-life Examples
- Understanding Hydration in SSR with React 18
- Next JS vs Gatsby: which to choose in 2024
- Deep Dive into Static vs Dynamic Rendering with NextJS
- Web Accessibility in React
- Vue JS vs React – CTO guideline in top frontend frameworks
- Create and publish ReactJS component on npm
- Use Async Actions Hook in ReactJS Web Development
- Web App vs Native App: what's better for you
Delve into these comprehensive resources to enhance your understanding of ReactJS and VueJS. If you’re interested in a collaboration involving frontend development with ReactJS or VueJS, please feel free to reach out to our sales team to discuss potential projects. For those looking to join our dynamic and skilled team of frontend developers, we encourage you to contact our recruitment department. We are always eager to welcome new talents who are passionate about pushing the boundaries of frontend technology. Let’s explore the possibilities of working together!
Did you like the article?Find out how we can help you.
Matt Sadowski
CEO of Mobile Reality

Related articles
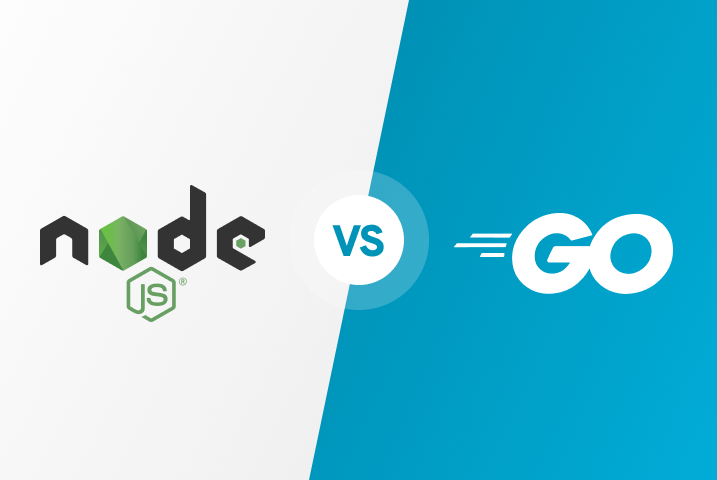
26.04.2024
GO vs Node JS : A Complete Comparison for CTOs
Discover the essential guide for CTOs comparing GO vs Node JS. Make the right choice for your tech stack. Get insights now! #node #nodejs #go #golang #CTO
Read full article
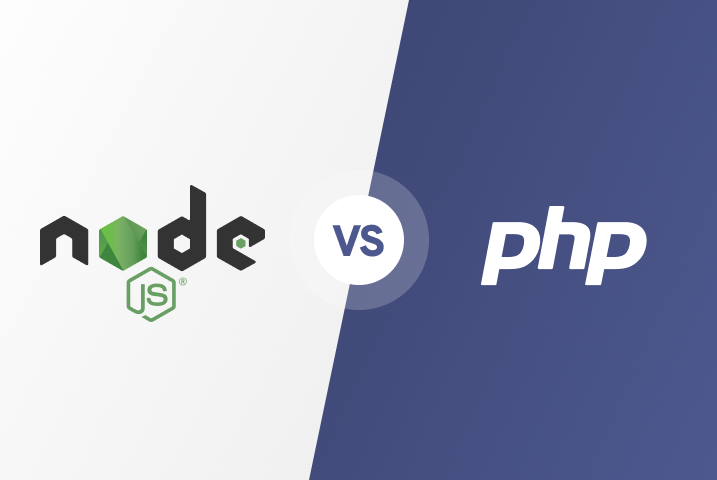
26.04.2024
Node JS vs PHP: A Complete Comparison for CTOs
Discover the essential guide for CTOs comparing Node JS vs PHP. Make the right choice for your tech stack. Get insights now! #nodejs #php #CTO
Read full article
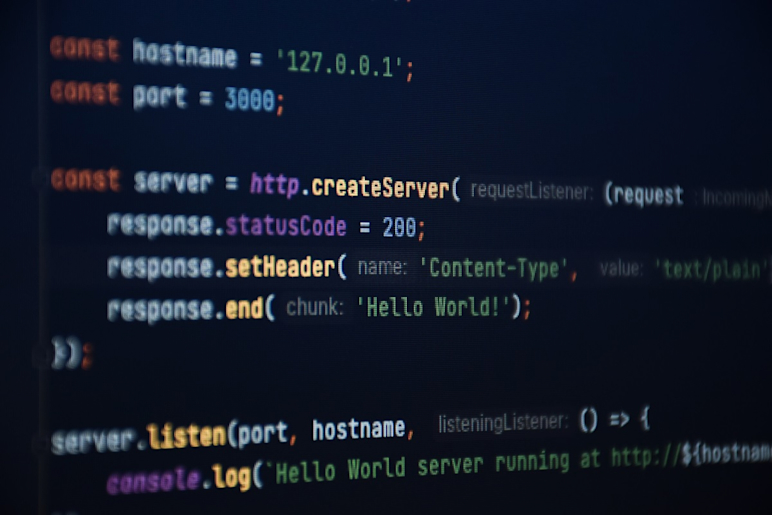
26.04.2024
Types of Apps You Can Build with Node JS in 2024
Read what types of applications you can build using Node JS technology.
Read full article